Encrypt/Decrypt a string on Linux using Openssl
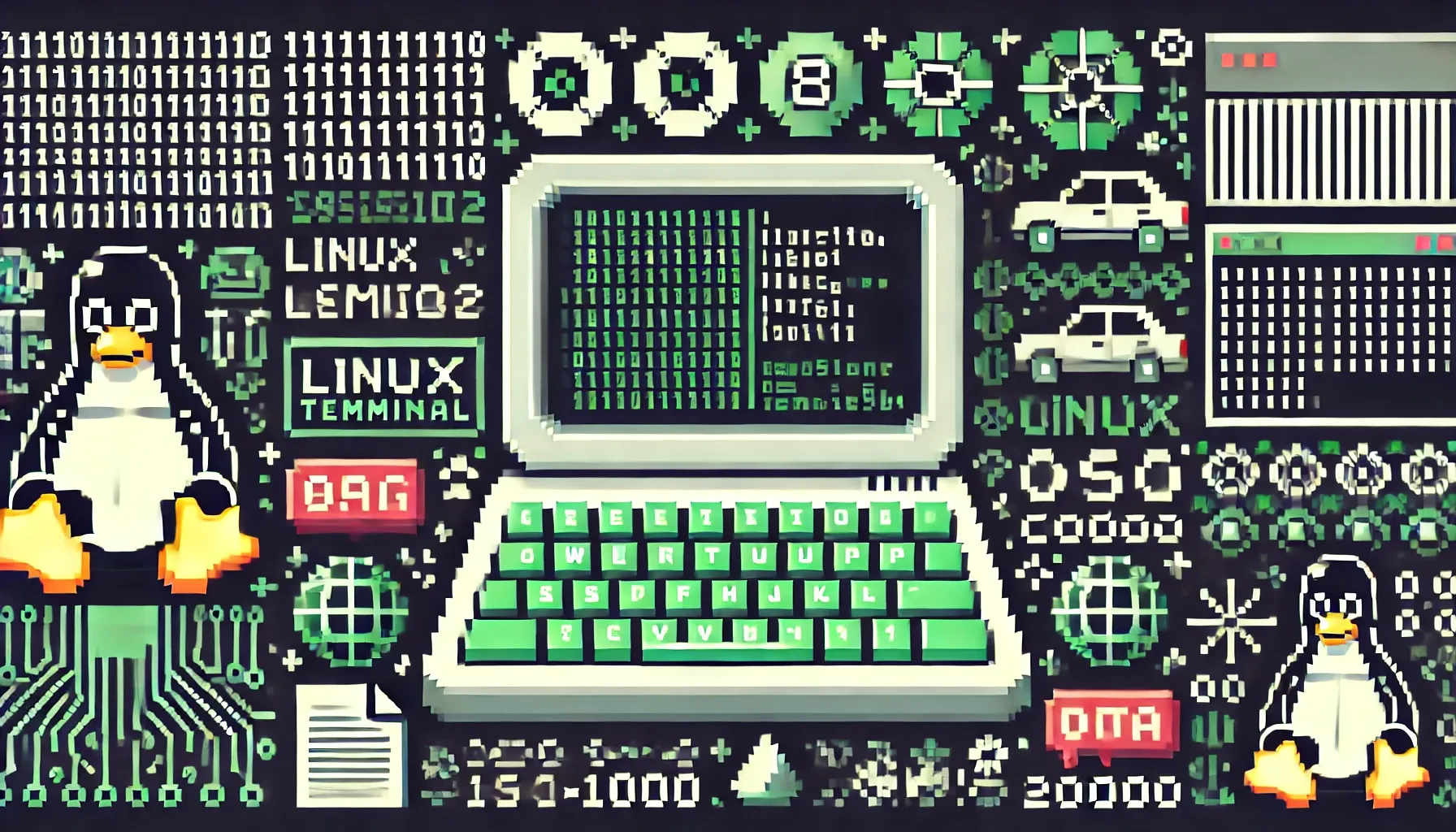
In this blog post I am going to show you how you can use Openssl to encrypt and decrypt a string on the command line in Linux.
Prerequisites
The prerequisite is that you have Openssl installed. Please read through this blog post on how to do this: https://www.howtoforge.com/tutorial/how-to-install-openssl-from-source-on-linux/
Encryption/Decryption commands
The encrpytion command for Openssl can be tested as follows:
echo "This is a sample string" | openssl enc -base64 -e -aes-256-cbc -salt -pass pass:SuperS3curePassw0rd! -pbkdf2
You will get a result similar to this: U2FsdGVkX197zphyvS6awkczRJrhl0i+uDbvAyde5TvCH7H+iavpguAx5BiY3Bx6
The encryption command for Openssl can be tested as follows:
echo U2FsdGVkX197zphyvS6awkczRJrhl0i+uDbvAyde5TvCH7H+iavpguAx5BiY3Bx6 | openssl enc -base64 -d -aes-256-cbc -salt -pass pass:SuperS3curePassw0rd! -pbkdf2
Run this command and you will get your decrypted string: This is a sample string
What you are doing here is to pipe a string to the Openssl command. Then you are defining:
- -base64: The output/input is a base64 string
- -e|-d: -e for encryption, -d for decryption
- -aes-256-cbc: The encryption/decryption algorhitm
- -salt: To add a salt to the encryption/decrpytion and make it more secure
- -pass: The password
- -pbkdf2: Method to derive a key from the password
Encryption/Decryption shell script on Linux using Openssl
You can download this script also from the Github repository:
git clone git@github.com:terencejackson8000/encrypt_decrypt.git
Or you can create your own shell script with the following content:
#!/usr/bin/env bash
#Get the parameteres of the script and assign them
while getopts m:s:p: flag
do
case "${flag}" in
m) mechanism=${OPTARG};;
s) string=${OPTARG};;
p) password=${OPTARG};;
esac
done
#Check if all parameters are set, if not show an error message and exit the script
if [ -z "$mechanism" ] || [ -z "$string" ] || [ -z "$password" ]
then echo "You need to set all variables to run the script: -m enc for encryption or dec for decryption, -s The string to encrypt/decrypt, -p The password for the encryption/decryption"
exit 0
fi
#if the mechanism is encryption => encrypt the string, if the mechanism is decryption => decrypt the string
if [ $mechanism == 'enc' ]
then
echo $string | openssl enc -base64 -e -aes-256-cbc -salt -pass pass:$password -pbkdf2
elif [ $mechanism == 'dec' ]
then
echo $string | openssl enc -base64 -d -aes-256-cbc -salt -pass pass:$password -pbkdf2
else
echo "Mechanism (-m) must be enc for encryption or dec for decryption"
fi
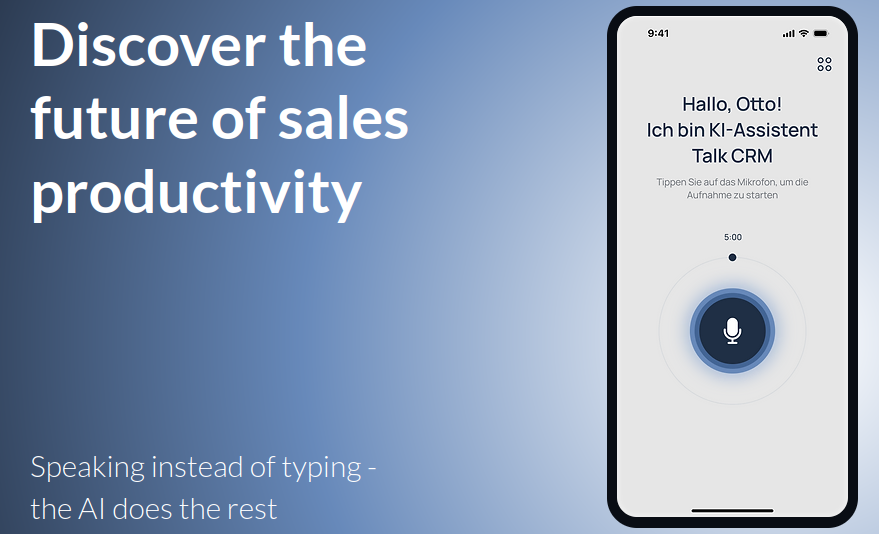
Next you have to make the script executable:
sudo chmod -x encrypt_decrypt.sh
And then you can run an ecryption:
./encrypt_decrypt.sh -m enc -s "This is a sample string" -p SuperS3curePassw0rd!
Or a decryption:
./encrypt_decrypt.sh -m dev -s "U2FsdGVkX1/mgl7Z+Y1cmNATJD/CnTHEFLlKhEwwUlpw8YYchYDoTAzMFGI20bIR" -p SuperS3curePassw0rd!